Api reference
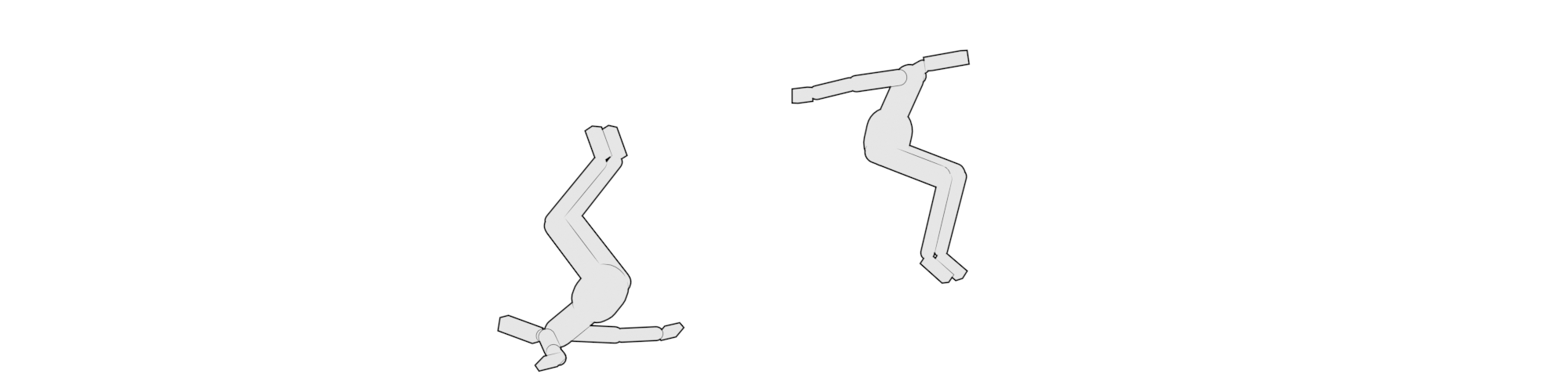
API Member Reference
The complete Ragdoll API.
from maya import cmds
import ragdoll.api as rd
box = cmds.polyCube()
solver = rd.createSolver()
marker = rd.assignMarker(box[0], solver)
rd.recordPhysics()
Function | Description |
---|---|
createSolver | Create a new rdSolver node |
createGroup | Create a new group under solver with name and opts |
assignMarkers | Assign markers to transforms belonging to solver |
assignMarker | Convenience function for passing and recieving a single transform |
createDistanceConstraint | Create a new distance constraint between parent and child |
createPinConstraint | Create a new constraint for parent and optionally parent |
createFixedConstraint | Create a new fixed constraint between parent and child |
linkSolver | Link solver a with b |
unlinkSolver | Unlink solver |
retargetMarker | Retarget marker to transform |
untargetMarker | Remove parent from child |
reparentMarker | Make new_parent the new parent of child |
unparentMarker | Remove parent from child |
replaceMesh | Replace the 'Mesh' shape type in marker with mesh . |
assignCollisionGroup | Assign markers to a new collision group |
addToCollisionGroup | Assign markers to a new collision group |
removeFromCollisionGroup | Assign markers to a new collision group |
exportPhysics | Export everything Ragdoll-related into fname |
importPhysics | Recreate Maya scene from exported Ragdoll file |
updatePhysics | Update existing physics from an exported Ragdoll file |
recordPhysics | Transfer simulation from solver to animation land |
reinterpretPhysics | Recreate Maya scene from exported Ragdoll file |
loadPhysics | Create a Maya scene from an exported Ragdoll file |
extractPhysics | Generate an animated joint hierarchy from solver |
deletePhysics | Delete Ragdoll from anything related to nodes |
deleteAllPhysics | Nuke it from orbit |
Argument Signatures
A more in-depth view on each function.
createSolver
Create a new rdSolver node
def createSolver(name, opts):
"""Create a new rdSolver node
____
/ \
/ | |
---/----\____/
/ /
---/-------/---
/ /
The solver is where the magic happens. Markers are connected to it
and solved within; populating its .outputMatrix attribute with the
final result.
Arguments:
name (str, optional): Override the default name of this solver
opts (dict, optional): Configure the solver with these options
Options:
frameskipMethod (int): Method to use whenever a frame is skipped,
can be either api.FrameskipPause or api.FrameskipIgnore
"""
createGroup
Create a new group under solver
with name
and opts
def createGroup(solver, opts):
"""Create a new group under `solver` with `name` and `opts`
Arguments:
solver (rdSolver): Owning solver of this group
name (str, optional): A custom name for this group
opts (dict, optional): Default attributes of this group
Options:
selfCollide (bool): Whether or not members of this
group should collide with each other.
"""
assignMarkers
Assign markers to transforms
belonging to solver
def assignMarkers(transforms, solver, opts):
"""Assign markers to `transforms` belonging to `solver`
Each marker transfers the translation and rotation of each transform
and generates its physical equivalent, ready for recording.
Arguments:
transforms (list): One or more transforms to assign markers onto
solver (rdSolver or rdGroup): Add newly created markers to this solver
opts (dict, optional): Options, see below
Options:
autoLimit (bool): Transfer locked channels into physics limits
preventIdenticalMarker (bool): Skip transforms with identical position
and orientation, typically sub-controls.
defaults (dict): Key/value pairs of default attribute values
"""
assignMarker
Convenience function for passing and recieving a single transform
def assignMarker(transform, solver, opts):
"""Convenience function for passing and recieving a single `transform`
"""
createDistanceConstraint
Create a new distance constraint between parent
and child
def createDistanceConstraint(parent, child, opts):
"""Create a new distance constraint between `parent` and `child`
"""
createPinConstraint
Create a new constraint for parent
and optionally parent
def createPinConstraint(child, parent, opts):
"""Create a new constraint for `parent` and optionally `parent`
Arguments:
child (rdMarker): Affected Marker
parent (rdMarker, optional): Attach child to parent, otherwise to world
transform (dagNode, optional): Parent the constraint shape node here
opts (dict, optional): User options
"""
createFixedConstraint
Create a new fixed constraint between parent
and child
def createFixedConstraint(parent, child, opts):
"""Create a new fixed constraint between `parent` and `child`
"""
linkSolver
Link solver a
with b
def linkSolver(a, b, opts):
"""Link solver `a` with `b`
This will make `a` part of `b`, allowing markers to interact.
Arguments:
a (rdSolver): The "child" solver
b (rdSolver): The "parent" solver
Returns:
Nothing
"""
unlinkSolver
Unlink solver
def unlinkSolver(solver, opts):
"""Unlink `solver`
From any other solver it may be connected to.
Arguments:
a (rdSolver): The solver to unlink from any other solver
Returns:
Nothing
"""
retargetMarker
Retarget marker
to transform
def retargetMarker(marker, transform, opts):
"""Retarget `marker` to `transform`
When recording, write simulation from `marker` onto `transform`,
regardless of where it is assigned.
"""
untargetMarker
Remove parent from child
def untargetMarker(marker, opts):
"""Remove parent from `child`
Meaning `child` will be a free marker, without a parent.
"""
reparentMarker
Make new_parent
the new parent of child
def reparentMarker(child, parent, opts):
"""Make `new_parent` the new parent of `child`
Arguments:
child (rdMarker): The marker whose about to have its parent changed
new_parent (rdMarker): The new parent of `child`
"""
unparentMarker
Remove parent from child
def unparentMarker(child, opts):
"""Remove parent from `child`
Meaning `child` will be a free marker, without a parent.
"""
replaceMesh
Replace the 'Mesh' shape type in marker
with mesh
.
def replaceMesh(marker, mesh, opts):
"""Replace the 'Mesh' shape type in `marker` with `mesh`.
Arguments:
marker (cmdx.Node): Rigid whose mesh to replace
mesh (cmdx.Node): Mesh to replace with
Returns:
Nothing
"""
assignCollisionGroup
Assign markers
to a new collision group
def assignCollisionGroup(markers, group, delete_orphans):
"""Assign `markers` to a new collision `group`
Arguments:
markers (list): Markers to assign
group (rdCollisionGroup, optional): The group to add Markers to,
if none is given a new one is created.
delete_orphans (bool, optional): Delete any orphaned groups
"""
addToCollisionGroup
Assign markers
to a new collision group
def addToCollisionGroup(markers, group, delete_orphans):
"""Assign `markers` to a new collision `group`
Arguments:
markers (list): Markers to assign
group (rdCollisionGroup, optional): The group to add Markers to,
if none is given a new one is created.
delete_orphans (bool, optional): Delete any orphaned groups
"""
removeFromCollisionGroup
Assign markers
to a new collision group
def removeFromCollisionGroup(markers, group, delete_orphans):
"""Assign `markers` to a new collision `group`
Arguments:
markers (list): Markers to assign
group (rdCollisionGroup, optional): The group to add Markers to,
if none is given a new one is created.
delete_orphans (bool, optional): Delete any orphaned groups
"""
exportPhysics
Export everything Ragdoll-related into fname
def exportPhysics(fname, opts):
"""Export everything Ragdoll-related into `fname`
Arguments:
fname (str, optional): Write to this file,
or console if no file is provided
data (dict, optional): Export this dictionary instead
Returns:
data (dict): Exported data as a dictionary
"""
importPhysics
Recreate Maya scene from exported Ragdoll file
def importPhysics(fname, opts):
"""Recreate Maya scene from exported Ragdoll file
User-interface attributes like `density` and display settings
are restored from a file otherwise mostly contains the raw
simulation data.
"""
updatePhysics
Update existing physics from an exported Ragdoll file
def updatePhysics(fname, opts):
"""Update existing physics from an exported Ragdoll file
"""
recordPhysics
Transfer simulation from solver
to animation land
def recordPhysics(solver, opts):
"""Transfer simulation from `solver` to animation land
Options:
start_time (int, optional): Record from this time
end_time (int, optional): Record to this time
include (list, optional): Record these transforms only
exclude (list, optional): Do not record these transforms
kinematic (bool, optional): Record kinematic frames too
maintain_offset (bool, optional): Maintain whatever offset is
between the source and destination transforms, default
value is True
"""
reinterpretPhysics
Recreate Maya scene from exported Ragdoll file
def reinterpretPhysics(fname, opts):
"""Recreate Maya scene from exported Ragdoll file
User-interface attributes like `density` and display settings
are restored from a file otherwise mostly contains the raw
simulation data.
"""
loadPhysics
Create a Maya scene from an exported Ragdoll file
def loadPhysics(fname, opts):
"""Create a Maya scene from an exported Ragdoll file
New transforms are generated and then assigned Markers.
"""
extractPhysics
Generate an animated joint hierarchy from solver
def extractPhysics(solver, opts):
"""Generate an animated joint hierarchy from `solver`
This will generate a new joint hierarchy and apply the full
simulation as keyframes onto it, for a complete replica of
the simulation in a Ragdoll-independent way.
"""
deletePhysics
Delete Ragdoll from anything related to nodes
def deletePhysics(nodes):
"""Delete Ragdoll from anything related to `nodes`
This will delete anything related to Ragdoll from your scenes, including
any attributes added (polluted) onto your animation controls.
Arguments:
nodes (list): Delete physics from these nodes
dry_run (bool, optional): Do not actually delete anything,
but still run through the process and throw exceptions
if any, and still return the results of what *would*
have been deleted if it wasn't dry.
"""
deleteAllPhysics
Nuke it from orbit
def deleteAllPhysics():
"""Nuke it from orbit
Return to simpler days, days before physics, with this one command.
"""
Constants
Some functions take constants for arguments.
from ragdoll import api
api.assign_marker(a, b, opts={"density": api.DensityFlesh})
Constant | Value |
---|---|
FrameskipPause | 0 |
FrameskipIgnore | 1 |
DisplayDefault | 0 |
DisplayWire | 1 |
DisplayConstant | 2 |
DisplayShaded | 3 |
DisplayMass | 4 |
DisplayFriction | 5 |
DisplayRestitution | 6 |
DisplayVelocity | 7 |
DisplayContacts | 8 |
InputInherit | 0 |
InputKinematic | 2 |
InputDynamic | 3 |
PGSSolverType | 0 |
TGSSolverType | 1 |
BoxShape | 0 |
SphereShape | 1 |
CapsuleShape | 2 |
MeshShape | 4 |
ConvexHullShape | 4 |
DensityOff | 0 |
DensityCotton | 1 |
DensityWood | 2 |
DensityFlesh | 3 |
DensityUranium | 4 |
DensityBlackHole | 5 |
DensityCustom | 6 |
StartTimeRangeStart | 0 |
StartTimeAnimationStart | 1 |
StartTimeCustom | 2 |
Lod0 | 0 |
Lod1 | 1 |
Lod2 | 2 |
LodCustom | 3 |
MatchByName | 0 |
MatchByHierarchy | 1 |
RecordConstraintMethod | 0 |
RecordMatchMethod | 1 |
InputOff | 3 |
InputGuide | 3 |